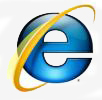
Random Number Generator in JavaScript
After searching the web for a simple random number generator, all I found were a bunch of specific random number generators in javaScript that were nice, but nothing that was generic enough to be used on a wide scale. Sure you can always use the "random()" script in javascript, but most developers have to write a chunck of code to make something useful. Well... I've created a generic function that will take most of the headache out of generating a random number. Check it out!
The idea here was to create generic number randomizer that would create a random whole number and would allow for exemptions.
What it does:
It will take whatever number you pass it and randomly generate a number from 0 to that number. It also allows the developer to add exemptions.
Options:
- MaxNumber: // the maximum number to which the randomizer will limit it's randomly generated numbers.
- Exempt: // numbers that you want the randomizer to ignore
How to call it:
randomizeMe(this, {maxNumber: 10, exempt: [2, 5]});
The result will be a random number between 0 and 10, excluding numbers 2 and 5.
Example:
Click here to try me out
(Alert a random number between 0 and 10 excluding 2 and 5)
Here is the code:
/* BEGIN: [Number Randomizer v.1 :: Author: Addam Driver :: Date: 5/5/2008 ] ** Disclaimer: Use at your own risk, copyright Addam Driver 2008 ** Example on calling this function: ** randomizeMe(this, {maxNumber: 10, exempt: [5, 6,7,8]}); ** The Randomizer randomly generate a number from 0 to the "maximumNumber" you pass it. ** If it comes across any exempt numbers, it will start the script over until it finds one that doesn't match the exempt. */ function randomizeMe(me, options){ var options = options || {}; // setup the options var imExempt = options.exempt || null; // capture the exempt numbers var maxNum = options.maxNumber || 0; // capture the maximum number to randomize var rand_no = Math.floor(Math.random()*maxNum); // run the random numbers if(imExempt != null){ // check to see if there are any exempt numbers imExempt = imExempt.toString(); // turn the exemptions into a string for(i=0; i <= maxNum; i++){ // loop throught the set number if(imExempt.search(rand_no) != -1){ // check for exempt numbers return randomizeMe(me, {exempt: imExempt, maxNumber: maxNum}); // start over } } } return rand_no;// return the new random number } /* END: [Number Randomizer] */
This script is expandable, so we can add to it and it will degrade gracefully as time goes on without having to change the way we call the script as time goes on.
I hope this helps someone out there! Cheers!